Cómo encontrar la transposición de una matriz en varios idiomas
La transposición de una matriz se obtiene intercambiando las filas y columnas de la matriz original. En este artículo, aprenderá a encontrar la transposición de una matriz cuadrada y rectangular usando C ++, Python, JavaScript y C.
Planteamiento del problema
Te dan un tapete de matriz [] [] . Necesita encontrar e imprimir la transposición de la matriz.
Ejemplos:
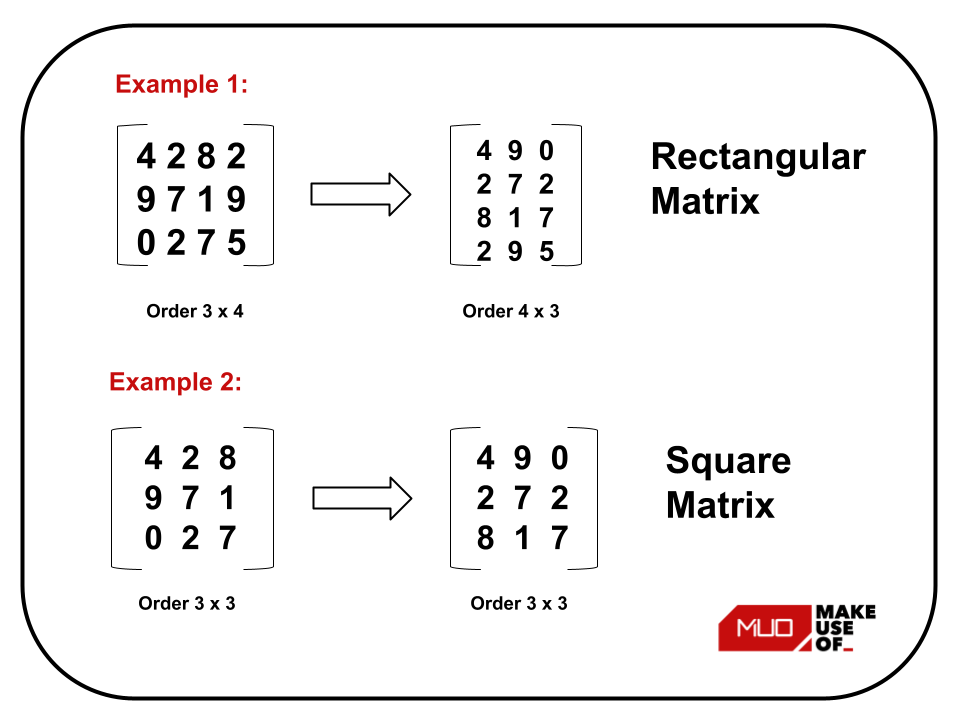
Cómo encontrar la transposición de una matriz rectangular
- El orden de la transposición de una matriz rectangular es opuesto al de la matriz original. Por ejemplo, si el orden de la matriz original es 3 x 4, entonces el orden de la transposición de esta matriz sería 4 x 3.
- Almacene cada columna de la matriz original como filas en la matriz transpuesta, es decir, transposeMatrix [i] [j] = mat [j] [i].
Programa C ++ para encontrar la transposición de una matriz rectangular
A continuación se muestra el programa C ++ para encontrar la transposición de una matriz rectangular:
// C++ program to find the transpose of a rectangular Matrix
#include <iostream>
using namespace std;
// The order of the initial matrix is 3 x 4
#define size1 3
#define size2 4
// Function to transpose a Matrix
void transposeMatrix(int mat[][size2], int transposeMatrix[][size1])
{
for (int i=0; i<size2; i++)
{
for (int j=0; j<size1; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size1][size2] = { {4, 2, 8, 2},
{9, 7, 1, 9},
{0, 2, 7, 5} };
cout << "Initial Matrix:" << endl;
// Printing the initial Matrix
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat
int transposedMatrix[size2][size1];
transposeMatrix(mat, transposedMatrix);
cout << "Transposed Matrix:" << endl;
// Printing the transposed Matrix
for (int i = 0; i < size2; i++)
{
for (int j = 0; j < size1; j++)
{
cout << transposedMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Producción:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Programa Python para encontrar la transposición de una matriz rectangular
A continuación se muestra el programa Python para encontrar la transposición de una matriz rectangular:
# Python program to find the transpose of a rectangular Matrix
# The order of the initial matrix is 3 x 4
size1 = 3
size2 = 4
# Function to transpose a Matrix
def transposeMatrix(mat, transposedMatrix):
for i in range(size2):
for j in range(size1):
transposedMatrix[i][j] = mat[j][i]
# Driver Code
mat = [ [4, 2, 8, 2],
[9, 7, 1, 9],
[0, 2, 7, 5] ]
print("Initial Matrix:")
# Printing the initial Matrix
for i in range(size1):
for j in range(size2):
print(mat[i][j], end=' ')
print()
# Variable to store the transposed Matrix
# The dimensions of transposedMatrix are opposite to that of mat
transposedMatrix = [[0 for x in range(size1)] for y in range(size2)]
transposeMatrix(mat, transposedMatrix)
print("Transposed Matrix:")
# Printing the transposed Matrix
for i in range(size2):
for j in range(size1):
print(transposedMatrix[i][j], end=' ')
print()
Producción:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Programa JavaScript para encontrar la transposición de una matriz rectangular
A continuación se muestra el programa JavaScript para encontrar la transposición de una matriz rectangular:
// JavaScript program to find the transpose of a rectangular Matrix
// The order of the initial matrix is 3 x 4
var size1 = 3
var size2 = 4
// Function to transpose a Matrix
function transposeMatrix(mat, transposeMatrix) {
for (let i=0; i<size2; i++) {
for (let j=0; j<size1; j++) {
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
var mat = [ [4, 2, 8, 2],
[9, 7, 1, 9],
[0, 2, 7, 5] ];
document.write("Initial Matrix:" + "<br>");
// Printing the initial Matrix
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
var transposedMatrix = new Array(size2);
for (let k = 0; k < size2; k++) {
transposedMatrix[k] = new Array(size1);
}
transposeMatrix(mat, transposedMatrix);
document.write("Transposed Matrix:" + "<br>");
// Printing the transposed Matrix
for (let i = 0; i < size2; i++) {
for (let j = 0; j < size1; j++) {
document.write(transposedMatrix[i][j] + " ");
}
document.write("<br>");
}
Producción:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Programa C para encontrar la transposición de una matriz rectangular
A continuación se muestra el programa en C para encontrar la transposición de una matriz rectangular:
// C program to find the transpose of a rectangular Matrix
#include <stdio.h>
// The order of the initial matrix is 3 x 4
#define size1 3
#define size2 4
// Function to transpose a Matrix
void transposeMatrix(int mat[][size2], int transposeMatrix[][size1])
{
for (int i=0; i<size2; i++)
{
for (int j=0; j<size1; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size1][size2] = { {4, 2, 8, 2},
{9, 7, 1, 9},
{0, 2, 7, 5} };
printf("Initial Matrix: n");
// Printing the initial Matrix
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
int transposedMatrix[size2][size1];
transposeMatrix(mat, transposedMatrix);
printf("Transposed Matrix: n");
// Printing the transposed Matrix
for (int i = 0; i < size2; i++)
{
for (int j = 0; j < size1; j++)
{
printf("%d ", transposedMatrix[i][j]);
}
printf("n");
}
return 0;
}
Producción:
Initial Matrix:
4 2 8 2
9 7 1 9
0 2 7 5
Transposed Matrix:
4 9 0
2 7 2
8 1 7
2 9 5
Cómo encontrar la transposición de una matriz cuadrada
- El orden de transposición de una matriz cuadrada es el mismo que el de la matriz original. Por ejemplo, si el orden de la matriz original es 3 x 3, el orden de la transposición de esta matriz aún sería 3 x 3. Por lo tanto, declare una matriz con el mismo orden que la matriz original.
- Almacene cada columna de la matriz original como filas en la matriz transpuesta, es decir, transposeMatrix [i] [j] = mat [j] [i].
Programa C ++ para encontrar la transposición de una matriz cuadrada
A continuación se muestra el programa C ++ para encontrar la transposición de una matriz cuadrada:
// C++ program to find the transpose of a square matrix
#include <iostream>
using namespace std;
// The order of the matrix is 3 x 3
#define size 3
// Function to transpose a Matrix
void transposeMatrix(int mat[][size], int transposeMatrix[][size])
{
for (int i=0; i<size; i++)
{
for (int j=0; j<size; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
int main()
{
int mat[size][size] = { {4, 2, 8},
{9, 7, 1},
{0, 2, 7} };
cout << "Initial Matrix:" << endl;
// Printing the initial Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
// Variable to store the transposed Matrix
int transposedMatrix[size][size];
transposeMatrix(mat, transposedMatrix);
cout << "Transposed Matrix:" << endl;
// Printing the transposed Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout << transposedMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Producción:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programa Python para encontrar la transposición de una matriz cuadrada
A continuación se muestra el programa Python para encontrar la transposición de una matriz cuadrada:
# Python program to find the transpose of a square Matrix
# The order of the initial matrix is 3 x 3
size = 3
# Function to transpose a Matrix
def transposeMatrix(mat, transposedMatrix):
for i in range(size):
for j in range(size):
transposedMatrix[i][j] = mat[j][i]
# Driver Code
mat = [ [4, 2, 8],
[9, 7, 1],
[0, 2, 7] ]
print("Initial Matrix:")
# Printing the initial Matrix
for i in range(size):
for j in range(size):
print(mat[i][j], end=' ')
print()
# Variable to store the transposed Matrix
transposedMatrix = [[0 for x in range(size)] for y in range(size)]
transposeMatrix(mat, transposedMatrix)
print("Transposed Matrix:")
# Printing the transposed Matrix
for i in range(size):
for j in range(size):
print(transposedMatrix[i][j], end=' ')
print()
Producción:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programa JavaScript para encontrar la transposición de una matriz cuadrada
A continuación se muestra el programa JavaScript para encontrar la transposición de una matriz cuadrada:
// JavaScript program to find the transpose of a square Matrix
// The order of the initial matrix is 3 x 3
var size = 3
// Function to transpose a Matrix
function transposeMatrix(mat, transposeMatrix) {
for (let i=0; i<size; i++) {
for (let j=0; j<size; j++) {
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
var mat = [ [4, 2, 8],
[9, 7, 1],
[0, 2, 7] ];
document.write("Initial Matrix:" + "<br>");
// Printing the initial Matrix
for (let i = 0; i < size; i++) {
for (let j = 0; j < size; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
// Variable to store the transposed Matrix
// The dimensions of transposedMatrix are opposite to that of mat1
var transposedMatrix = new Array(size);
for (let k = 0; k < size; k++) {
transposedMatrix[k] = new Array(size);
}
transposeMatrix(mat, transposedMatrix);
document.write("Transposed Matrix:" + "<br>");
// Printing the transposed Matrix
for (let i = 0; i < size; i++) {
for (let j = 0; j < size; j++) {
document.write(transposedMatrix[i][j] + " ");
}
document.write("<br>");
}
Producción:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Programa C para encontrar la transposición de una matriz cuadrada
A continuación se muestra el programa en C para encontrar la transposición de una matriz cuadrada:
// C program to find the transpose of a square Matrix
#include <stdio.h>
// The order of the initial matrix is 3 x 3
#define size 3
// Function to transpose a Matrix
void transposeMatrix(int mat[][size], int transposeMatrix[][size])
{
for (int i=0; i<size; i++)
{
for (int j=0; j<size; j++)
{
transposeMatrix[i][j] = mat[j][i];
}
}
}
// Driver Code
int main()
{
int mat[size][size] = { {4, 2, 8},
{9, 7, 1},
{0, 2, 7} };
printf("Initial Matrix: n");
// Printing the initial Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
// Variable to store the transposed Matrix
int transposedMatrix[size][size];
transposeMatrix(mat, transposedMatrix);
printf("Transposed Matrix: n");
// Printing the transposed Matrix
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
printf("%d ", transposedMatrix[i][j]);
}
printf("n");
}
return 0;
}
Producción:
Initial Matrix:
4 2 8
9 7 1
0 2 7
Transposed Matrix:
4 9 0
2 7 2
8 1 7
Resolver problemas básicos de programación basados en matrices
Una matriz es una cuadrícula que se utiliza para almacenar o mostrar datos en un formato estructurado. Las matrices se utilizan ampliamente en programación para realizar diversas operaciones. Si está buscando cubrir todas las bases de entrevistas de codificación, debe saber cómo realizar operaciones básicas como suma, resta, multiplicación y más en matrices.