Cómo comprobar si dos matrices son idénticas a la programación
Se dice que dos matrices son idénticas si ambas tienen el mismo número de filas, columnas y los mismos elementos correspondientes. En este artículo, aprenderá a verificar si dos matrices son idénticas usando Python, C ++, JavaScript y C.
Planteamiento del problema
Se le dan dos matrices mat1 [] [] y mat2 [] [] . Debe verificar si las dos matrices son idénticas. Si las dos matrices son idénticas, escriba "Sí, las matrices son idénticas". Y si las dos matrices no son idénticas, escriba "No, las matrices no son idénticas".
Ejemplos :
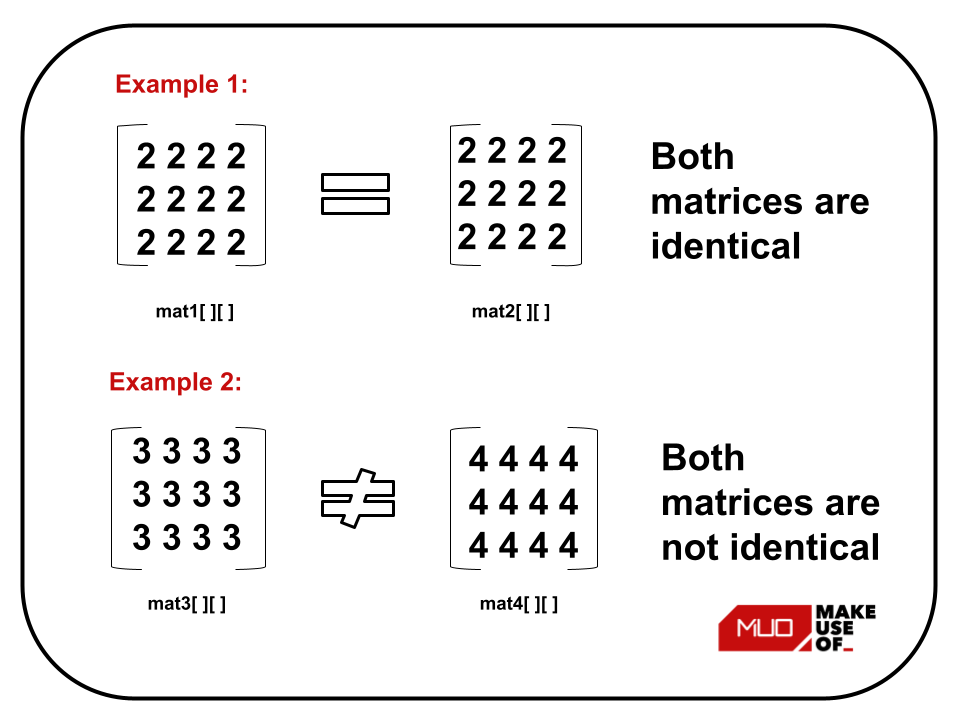
Condición para que dos matrices sean idénticas
Se dice que dos matrices son idénticas si y solo si satisfacen las siguientes condiciones:
- Ambas matrices tienen el mismo número de filas y columnas.
- Ambas matrices tienen los mismos elementos correspondientes.
Enfoque para comprobar si las dos matrices dadas son idénticas
Puede seguir el enfoque a continuación para verificar si las dos matrices dadas son idénticas o no:
- Ejecute un ciclo anidado para atravesar cada elemento de ambas matrices.
- Si alguno de los elementos correspondientes de las dos matrices no es igual, devuelve falso.
- Y si no se encuentran elementos correspondientes diferentes 'hasta el final del ciclo, devuelve verdadero.
Programa C ++ para comprobar si las dos matrices dadas son idénticas
A continuación se muestra el programa C ++ para verificar si las dos matrices dadas son idénticas o no:
// C++ program to check if two matrices are identical
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << mat[i][j] << " ";
}
cout << endl;
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 1:" << endl;
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
cout << "Matrix 2:" << endl;
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
cout << "Matrix 3:" << endl;
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
cout << "Matrix 4:" << endl;
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
cout << "Yes, the matrices are identical" << endl;
}
else
{
cout << "No, the matrices are not identical" << endl;
}
return 0;
}
Producción:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Programa Python para verificar si las dos matrices dadas son idénticas
A continuación se muestra el programa Python para verificar si las dos matrices dadas son idénticas o no:
# Python program to check if two matrices are identical
# The order of the matrix is 3 x 4
size1 = 3
size2 = 4
# Function to check if two matrices are identical
def isIdentical(mat1, mat2):
for i in range(size1):
for j in range(size2):
if (mat1[i][j] != mat2[i][j]):
return False
return True
# Function to print a matrix
def printMatrix(mat):
for i in range(size1):
for j in range(size2):
print(mat[i][j], end=' ')
print()
# Driver code
# 1st Matrix
mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 1:")
printMatrix(mat1)
# 2nd Matrix
mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ]
print("Matrix 2:")
printMatrix(mat2)
if (isIdentical(mat1, mat2)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
# 3rd Matrix
mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ]
print("Matrix 3:")
printMatrix(mat3)
# 4th Matrix
mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ]
print("Matrix 4:")
printMatrix(mat4)
if (isIdentical(mat3, mat4)):
print("Yes, the matrices are identical")
else:
print("No, the matrices are not identical")
Producción:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Programa JavaScript para comprobar si las dos matrices dadas son idénticas
A continuación se muestra el programa JavaScript para verificar si las dos matrices dadas son idénticas o no:
// JavaScript program to check if two matrices are identical
// The order of the matrix is 3 x 4
var size1 = 3;
var size2 = 4;
// Function to check if two matrices are identical
function isIdentical(mat1, mat2) {
for (let i = 0; i < size1; i++)
{
for (let j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
function printMatrix(mat) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(mat[i][j] + " ");
}
document.write("<br>");
}
}
// Driver code
// 1st Matrix
var mat1 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 1:" + "<br>");
printMatrix(mat1);
// 2nd Matrix
var mat2 = [ [2, 2, 2, 2],
[2, 2, 2, 2],
[2, 2, 2, 2] ];
document.write("Matrix 2:" + "<br>");
printMatrix(mat2);
if (isIdentical(mat1, mat2)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
// 3rd Matrix
var mat3 = [ [3, 3, 3, 3],
[3, 3, 3, 3],
[3, 3, 3, 3] ];
document.write("Matrix 3:" + "<br>");
printMatrix(mat3);
// 4th Matrix
var mat4 = [ [4, 4, 4, 4],
[4, 4, 4, 4],
[4, 4, 4, 4] ];
document.write("Matrix 4:" + "<br>");
printMatrix(mat4);
if (isIdentical(mat3, mat4)) {
document.write("Yes, the matrices are identical" + "<br>");
} else{
document.write("No, the matrices are not identical" + "<br>");
}
Producción:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Programa C para comprobar si las dos matrices dadas son idénticas
A continuación se muestra el programa en C para verificar si las dos matrices dadas son idénticas o no:
// C program to check if two matrices are identical
#include <stdio.h>
#include <stdbool.h>
// The order of the matrix is 3 x 4
#define size1 3
#define size2 4
// Function to check if two matrices are identical
bool isIdentical(int mat1[][size2], int mat2[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
if (mat1[i][j] != mat2[i][j])
{
return false;
}
}
}
return true;
}
// Function to print a matrix
void printMatrix(int mat[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", mat[i][j]);
}
printf("n");
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 1:n");
printMatrix(mat1);
// 2nd Matrix
int mat2[size1][size2] = { {2, 2, 2, 2},
{2, 2, 2, 2},
{2, 2, 2, 2} };
printf("Matrix 2:n");
printMatrix(mat2);
if(isIdentical(mat1, mat2))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
// 3rd Matrix
int mat3[size1][size2] = { {3, 3, 3, 3},
{3, 3, 3, 3},
{3, 3, 3, 3} };
printf("Matrix 3: n");
printMatrix(mat3);
// 4th Matrix
int mat4[size1][size2] = { {4, 4, 4, 4},
{4, 4, 4, 4},
{4, 4, 4, 4} };
printf("Matrix 4: n");
printMatrix(mat4);
if(isIdentical(mat3, mat4))
{
printf("Yes, the matrices are identical n");
}
else
{
printf("No, the matrices are not identical n");
}
return 0;
}
Producción:
Matrix 1:
2 2 2 2
2 2 2 2
2 2 2 2
Matrix 2:
2 2 2 2
2 2 2 2
2 2 2 2
Yes, the matrices are identical
Matrix 3:
3 3 3 3
3 3 3 3
3 3 3 3
Matrix 4:
4 4 4 4
4 4 4 4
4 4 4 4
No, the matrices are not identical
Aprenda un nuevo lenguaje de programación
La informática se está expandiendo a un ritmo muy rápido y la competencia en este campo es más intensa que nunca. Debe mantenerse actualizado con las últimas habilidades y lenguajes de programación. Ya sea que sea un programador principiante o experimentado, en cualquier caso, debe aprender algunos de los lenguajes de programación de acuerdo con los requisitos de la industria.